Fully connected
Fully connected#
New in version 2.11.
#include <pagmo/topologies/fully_connected.hpp>
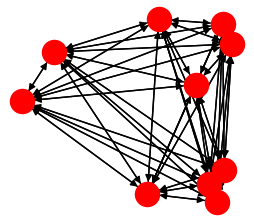
-
class fully_connected#
This user-defined topology (UDT) represents a complete graph (that is, a topology in which all vertices connect to all other vertices). The edge weight is configurable at construction, and it will be the same for all the edges in the topology.
-
fully_connected()#
Default constructor.
Equivalent to the constructor from edge weight with w = 1.
-
explicit fully_connected(double w)#
Constructor from edge weight.
Equivalent to the constructor from number of vertices n = 0 and edge weight w.
- Parameters
w – the weight of the edges.
- Throws
std::invalid_argument – if w is not in the \(\left[0, 1\right]\) range.
-
explicit fully_connected(std::size_t n, double w)#
Constructor from number of vertices and edge weight.
This constructor will initialise a
fully_connected
topology with n vertices and whose edges will all have a weight of w.- Parameters
n – the desired number of vertices.
w – the weight of the edges.
- Throws
std::invalid_argument – if w is not in the \(\left[0, 1\right]\) range.
-
fully_connected(const fully_connected&)#
-
fully_connected(fully_connected&&) noexcept#
fully_connected
is copy and move constructible.
-
void push_back()#
Add a new vertex.
-
std::pair<std::vector<std::size_t>, vector_double> get_connections(std::size_t i) const#
Get the list of connections to the i-th vertex.
- Parameters
i – the index of the vertex whose connections will be returned.
- Returns
the list of vertices connecting to the i-th vertex (that is, all vertices apart from i itself) and the corresponding edge weights.
- Throws
std::invalid_argument – if i is not smaller than the current size of the topology.
-
std::string get_name() const#
- Returns
"Fully connected"
.
-
std::string get_extra_info() const#
- Returns
a human-readable string containing additional info about this topology.
-
double get_weight() const#
- Returns
the weight w used when constructing this topology.
-
std::size_t num_vertices() const#
- Returns
the number of vertices in the topology.
-
bgl_graph_t to_bgl() const#
New in version 2.15.
Convert to a BGL graph.
Warning
The graph representation of a fully connected topology requires \(\operatorname{O}\left( n^2 \right)\) memory in the number of vertices. Be careful when invoking this function on large topologies.
- Returns
a complete graph representing
this
.- Throws
unspecified – any exception thrown by the public BGL API.
-
fully_connected()#