Algorithm class#
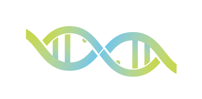
- class pygmo.algorithm(uda=null_algorithm())#
Algorithm class.
This class represents an optimization algorithm. An algorithm can be stochastic, deterministic, population based, derivative-free, using hessians, using gradients, a meta-heuristic, evolutionary, etc.. Via this class pygmo offers a common interface to all types of algorithms that can be applied to find solution to a generic mathematical programming problem as represented by the
problem
class.In order to define an optimizaztion algorithm in pygmo, the user must first define a class whose methods describe the properties of the algorithm and implement its logic. In pygmo, we refer to such a class as a user-defined algorithm, or UDA for short. Once defined and instantiated, a UDA can then be used to construct an instance of this class,
algorithm
, which provides a generic interface to optimization algorithms.Every UDA must implement at least the following method:
def evolve(self, pop): ...
The
evolve()
method takes as input apopulation
, and it is expected to return a new population generated by the evolution (or optimisation) of the original population.Additional optional methods can be implemented in a UDA:
def has_set_seed(self): ... def set_seed(self, s): ... def has_set_verbosity(self): ... def set_verbosity(self, l): ... def get_name(self): ... def get_extra_info(self): ...
See the documentation of the corresponding methods in this class for details on how the optional methods in the UDA should be implemented and on how they are used by
algorithm
. Note that the exposed C++ algorithms can also be used as UDAs, even if they do not expose any of the mandatory or optional methods listed above (see here for the full list of UDAs already coded in pygmo).This class is the Python counterpart of the C++ class
pagmo::algorithm
.- Parameters
uda – a user-defined algorithm, either C++ or Python
- Raises
NotImplementedError – if uda does not implement the mandatory method detailed above
unspecified – any exception thrown by methods of the UDA invoked during construction, the deep copy of the UDA, the constructor of the underlying C++ class, or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- evolve(pop)#
This method will invoke the
evolve()
method of the UDA. This is where the core of the optimization (evolution) is made.- Parameters
pop (
population
) – starting population- Returns
evolved population
- Return type
- Raises
unspecified – any exception thrown by the
evolve()
method of the UDA or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- extract(t)#
Extract the user-defined algorithm.
This method allows to extract a reference to the user-defined algorithm (UDA) stored within this
algorithm
instance. The behaviour of this function depends on the value of t (which must be atype
) and on the type of the internal UDA:if the type of the UDA is t, then a reference to the UDA will be returned (this mirrors the behaviour of the corresponding C++ method
pagmo::algorithm::extract()
),if t is
object
and the UDA is a Python object (as opposed to an exposed C++ algorithm), then a reference to the UDA will be returned (this allows to extract a Python UDA without knowing its type),otherwise,
None
will be returned.
- Parameters
t (
type
) – the type of the user-defined algorithm to extract- Returns
a reference to the internal user-defined algorithm, or
None
if the extraction fails- Raises
Examples
>>> import pygmo as pg >>> a1 = pg.algorithm(pg.compass_search()) >>> a1.extract(pg.compass_search) <pygmo.core.compass_search at 0x7f8e4792b670> >>> a1.extract(pg.de) is None True >>> class algo: ... def evolve(self, pop): ... return pop >>> a2 = pg.algorithm(algo()) >>> a2.extract(object) <__main__.algo at 0x7f8e478c04e0> >>> a2.extract(algo) <__main__.algo at 0x7f8e478c04e0> >>> a2.extract(pg.de) is None True
- get_extra_info()#
Algorithm’s extra info.
If the UDA provides a
get_extra_info()
method, then this method will return the output of itsget_extra_info()
method. Otherwise, an empty string will be returned.- Returns
extra info about the UDA
- Return type
- Raises
unspecified – any exception thrown by the
get_extra_info()
method of the UDA
- get_name()#
Algorithm’s name.
If the UDA provides a
get_name()
method, then this method will return the output of itsget_name()
method. Otherwise, an implementation-defined name based on the type of the UDA will be returned.- Returns
the algorithm’s name
- Return type
- get_thread_safety()#
Algorithm’s thread safety level.
This method will return a value of the enum
pygmo.thread_safety
which indicates the thread safety level of the UDA. Unlike in C++, in Python it is not possible to re-implement this method in the UDA. That is, for C++ UDAs, the returned value will be the value returned by theget_thread_safety()
method of the UDA. For Python UDAs, the returned value will be unconditionallynone
.- Returns
the thread safety level of the UDA
- Return type
a value of
pygmo.thread_safety
- has_set_seed()#
Check if the
set_seed()
method is available in the UDA.This method will return
True
if theset_seed()
method is available in the UDA,False
otherwise.The availability of the
set_seed()
method is determined as follows:if the UDA does not provide a
set_seed()
method, then this method will always returnFalse
;if the UDA provides a
set_seed()
method but it does not provide ahas_set_seed()
method, then this method will always returnTrue
;if the UDA provides both a
set_seed()
and ahas_set_seed()
method, then this method will return the output of thehas_set_seed()
method of the UDA.
The optional
has_set_seed()
method of the UDA must return abool
. For information on how to implement theset_seed()
method of the UDA, seeset_seed()
.- Returns
a flag signalling the availability of the
set_seed()
method in the UDA- Return type
bool
- has_set_verbosity()#
Check if the
set_verbosity()
method is available in the UDA.This method will return
True
if theset_verbosity()
method is available in the UDA,False
otherwise.The availability of the
set_verbosity()
method is determined as follows:if the UDA does not provide a
set_verbosity()
method, then this method will always returnFalse
;if the UDA provides a
set_verbosity()
method but it does not provide ahas_set_verbosity()
method, then this method will always returnTrue
;if the UDA provides both a
set_verbosity()
and ahas_set_verbosity()
method, then this method will return the output of thehas_set_verbosity()
method of the UDA.
The optional
has_set_verbosity()
method of the UDA must return abool
. For information on how to implement theset_verbosity()
method of the UDA, seeset_verbosity()
.- Returns
a flag signalling the availability of the
set_verbosity()
method in the UDA- Return type
bool
- is_(t)#
Check the type of the user-defined algorithm.
This method returns
False
ifextract(t)
returnsNone
, andTrue
otherwise.
- is_stochastic()#
Alias for
has_set_seed()
.
- set_seed(seed)#
Set the seed for the stochastic evolution.
This method will set the seed to be used in the
evolve()
method of the UDA for all stochastic variables. If the UDA provides aset_seed()
method, then itsset_seed()
method will be invoked. Otherwise, an error will be raised. The seed parameter must be non-negative.The
set_seed()
method of the UDA must be able to take anint
as input parameter.- Parameters
seed (
int
) – the random seed- Raises
NotImplementedError – if the UDA does not provide a
set_seed()
methodunspecified – any exception raised by the
set_seed()
method of the UDA or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- set_verbosity(level)#
Set the verbosity of logs and screen output.
This method will set the level of verbosity for the algorithm. If the UDA provides a
set_verbosity()
method, then itsset_verbosity()
method will be invoked. Otherwise, an error will be raised.The exact meaning of the input parameter level is dependent on the UDA.
The
set_verbosity()
method of the UDA must be able to take anint
as input parameter.- Parameters
level (
int
) – the desired verbosity level- Raises
NotImplementedError – if the UDA does not provide a
set_verbosity()
methodunspecified – any exception raised by the
set_verbosity()
method of the UDA or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)