Population class#
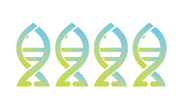
- class pygmo.population(prob=None, size=0, b=None, seed=None)#
The population class.
This class represents a population of individuals, i.e., potential candidate solutions to a given problem. In pygmo an individual is determined:
by a unique ID used to track him across generations and migrations,
by a chromosome (a decision vector),
by the fitness of the chromosome as evaluated by a
problem
and thus including objectives, equality constraints and inequality constraints if present.
A special mechanism is implemented to track the best individual that has ever been part of the population. Such an individual is called champion and its decision vector and fitness vector are automatically kept updated. The champion is not necessarily an individual currently in the population. The champion is only defined and accessible via the population interface if the
problem
currently contained in thepopulation
is single objective.See also the docs of the C++ class
pagmo::population
.- Parameters
prob – a user-defined problem (either Python or C++), or an instance of
problem
(if prob isNone
, a default-constructedproblem
will be used in its stead)size (
int
) – the number of individualsb – a user-defined batch fitness evaluator (either Python or C++), or an instance of
bfe
(if b isNone
, the evaluation of the population’s individuals will be performed in sequential mode)seed (
int
) – the random seed (if seed isNone
, a randomly-generated value will be used in its stead)
- Raises
TypeError – if size is not an
int
or seed is notNone
and not anint
OverflowError – is size or seed are negative
unspecified – any exception thrown by the invoked C++ constructors, by the constructor of
problem
, or the constructor ofbfe
, or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- best_idx(tol=self.problem.c_tol)#
Index of the best individual.
If the problem is single-objective and unconstrained, the best is simply the individual with the smallest fitness. If the problem is, instead, single objective, but with constraints, the best will be defined using the criteria specified in
pygmo.sort_population_con()
. If the problem is multi-objective one single best is not well defined. In this case the user can still obtain a strict ordering of the population individuals by calling thepygmo.sort_population_mo()
function.- Parameters
tol (
float
or array-like object) – scalar tolerance or vector of tolerances to be applied to each constraints. By default, thec_tol
attribute from the population’s problem is used.- Returns
the index of the best individual
- Return type
- Raises
ValueError – if the problem is multiobjective and thus a best individual is not well defined, or if the population is empty
unspecified – any exception thrown by
pagmo::sort_population_con()
- property champion_f#
Champion’s fitness vector.
This read-only property contains an array of
float
representing the fitness vector of the population’s champion.Note
If the problem is stochastic, the champion is the individual that had the lowest fitness for some lucky seed, not on average across seeds. Re-evaluating its decision vector may then result in a different fitness.
- Returns
the champion’s fitness vector
- Return type
1D NumPy float array
- Raises
ValueError – if the current problem is not single objective
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- property champion_x#
Champion’s decision vector.
This read-only property contains an array of
float
representing the decision vector of the population’s champion.Note
If the problem is stochastic the champion is the individual that had the lowest fitness for some lucky seed, not on average across seeds. Re-evaluating its decision vector may then result in a different fitness.
- Returns
the champion’s decision vector
- Return type
1D NumPy float array
- Raises
ValueError – if the current problem is not single objective
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_ID()#
This method will return the IDs of the individuals as a 1D NumPy array.
Each element of the returned array represents the ID of the individual at the corresponding position in the population.
- Returns
a deep copy of the IDs of the individuals
- Return type
1D NumPy int array
- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_f()#
This method will return the fitness vectors of the individuals as a 2D NumPy array.
Each row of the returned array represents the fitness vector of the individual at the corresponding position in the population.
- Returns
a deep copy of the fitness vectors of the individuals
- Return type
2D NumPy float array
- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_seed()#
This method will return the random seed of the population.
- Returns
the random seed of the population
- Return type
- get_x()#
This method will return the chromosomes of the individuals as a 2D NumPy array.
Each row of the returned array represents the chromosome of the individual at the corresponding position in the population.
- Returns
a deep copy of the chromosomes of the individuals
- Return type
2D NumPy float array
- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- property problem#
Population’s problem.
This read-only property gives direct access to the
problem
stored within the population.- Returns
a reference to the internal problem
- Return type
- push_back(x, f=None)#
Adds one decision vector (chromosome) to the population.
This method will append a new chromosome x to the population, creating a new unique identifier for the newly born individual and, if f is not provided, evaluating its fitness. If f is provided, the fitness of the new individual will be set to f. It is the user’s responsibility to ensure that f actually corresponds to the fitness of x.
In case of exceptions, the population will not be altered.
- Parameters
x (array-like object) – decision vector to be added to the population
- Raises
ValueError – if the dimensions of x or f (if provided) are incompatible with the population’s problem
unspecified – any exception thrown by
pygmo.problem.fitness()
or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- random_decision_vector()#
This method will create a random decision vector within the problem’s bounds.
- Returns
a random decision vector within the problem’s bounds
- Return type
- Raises
unspecified – any exception thrown by
pygmo.random_decision_vector()
- set_x(i, x)#
Sets the \(i\)-th individual decision vector.
Sets the chromosome of the \(i\)-th individual to the value x and changes its fitness accordingly. The individual’s ID remains the same.
Note
A call to this method triggers one fitness function evaluation.
- Parameters
i (
int
) – individual’s index in the populationx (array-like object) – a decision vector (chromosome)
- Raises
ValueError – if i is invalid, or if x has the wrong dimensions (i.e., the dimension is inconsistent with the problem’s properties)
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- set_xf(i, x, f)#
Sets the \(i\)-th individual decision vector, and fitness.
Sets simultaneously the \(i\)-th individual decision vector and fitness thus avoiding to trigger a fitness function evaluation.
Note
The user must make sure that the input fitness f makes sense as pygmo will only check its dimension.
- Parameters
i (
int
) – individual’s index in the populationx (array-like object) – a decision vector (chromosome)
f (array-like object) – a fitness vector
- Raises
ValueError – if i is invalid, or if x or f have the wrong dimensions (i.e., their dimensions are inconsistent with the problem’s properties)
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- worst_idx(tol=0.)#
Index of the worst individual.
If the problem is single-objective and unconstrained, the worst is simply the individual with the largest fitness. If the problem is, instead, single objective, but with constraints, the worst will be defined using the criteria specified in
pygmo.sort_population_con()
. If the problem is multi-objective one single worst is not well defined. In this case the user can still obtain a strict ordering of the population individuals by calling thepygmo.sort_population_mo()
function.- Parameters
tol (
float
or array-like object) – scalar tolerance or vector of tolerances to be applied to each constraints- Returns
the index of the worst individual
- Return type
- Raises
ValueError – if the problem is multiobjective and thus a worst individual is not well defined, or if the population is empty
unspecified – any exception thrown by
pygmo.sort_population_con()