Island class#
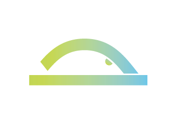
- class pygmo.island(**kwargs)#
Island class.
In the pygmo jargon, an island is a class that encapsulates the following entities:
a user-defined island (UDI),
an
algorithm
,a
population
,a replacement policy (of type
r_policy
),a selection policy (of type
s_policy
).
Through the UDI, the island class manages the asynchronous evolution (or optimisation) of its
population
via the algorithm’sevolve()
method. Depending on the UDI, the evolution might take place in a separate thread (e.g., if the UDI is athread_island
), in a separate process (e.g., if the UDI is amp_island
) or even in a separate machine (e.g., if the UDI is aipyparallel_island
). The evolution is always asynchronous (i.e., running in the “background”) and it is initiated by a call to theevolve()
method. At any time the user can query the state of the island and fetch its internal data members. The user can explicitly wait for pending evolutions to conclude by calling thewait()
andwait_check()
methods. The status of ongoing evolutions in the island can be queried via thestatus
attribute.The replacement and selection policies are used when the island is part of an
archipelago
. They establish how individuals are selected and replaced from the island when migration across islands occurs within thearchipelago
. If the island is not part of anarchipelago
, the replacement and selection policies play no role.Typically, pygmo users will employ an already-available UDI in conjunction with this class (see here for a full list), but advanced users can implement their own UDI types. A user-defined island must implement the following method:
def run_evolve(self, algo, pop): ...
The
run_evolve()
method of the UDI will use the inputalgorithm
’sevolve()
method to evolve the inputpopulation
and, once the evolution is finished, it will return the algorithm used for the evolution and the evolvedpopulation
.In addition to the mandatory
run_evolve()
method, a UDI may implement the following optional methods:def get_name(self): ... def get_extra_info(self): ...
See the documentation of the corresponding methods in this class for details on how the optional methods in the UDI are used by
island
.Note that, due to the asynchronous nature of
island
, a UDI has certain requirements regarding thread safety. Specifically,run_evolve()
is always called in a separate thread of execution, and consequently:multiple UDI objects may be calling their own
run_evolve()
method concurrently,in a specific UDI object, any method from the public API of the UDI may be called while
run_evolve()
is running concurrently in another thread. Thus, UDI writers must ensure that actions such as copying the UDI, calling the optional methods (such asget_name()
), etc. can be safely performed while the island is evolving.
An island can be initialised in a variety of ways using keyword arguments:
if the arguments list is empty, a default
island
is constructed, containing athread_island
UDI, anull_algorithm
algorithm, an empty population with problem typenull_problem
, and default-constructedr_policy
ands_policy
;if the arguments list contains algo, pop and, optionally, udi, r_pol and s_pol, then the constructor will initialise an
island
containing the specified algorithm, population, UDI and replacement/selection policies. If r_pol and/or s_pol are not supplied, the replacement/selection policies will be default-constructed. If the udi parameter is not supplied, the UDI type is chosen according to a heuristic which depends on the platform, the Python version and the supplied algo and pop parameters:if algo and pop’s problem provide at least the
basic
thread_safety
guarantee, thenthread_island
will be selected as UDI type;otherwise, if the current platform is Windows or the Python version is at least 3.4, then
mp_island
will be selected as UDI type, elseipyparallel_island
will be chosen;
if the arguments list contains algo, prob, size and, optionally, udi, b, seed, r_pol and s_pol, then a
population
will be constructed from prob, size, b and seed, and the construction will then proceed in the same way detailed above (i.e., algo and the newly-created population are used to initialise the island’s algorithm and population, the UDI, if not specified, will be chosen according to the heuristic detailed above, and the replacement/selection policies are given by r_pol and s_pol).
If the keyword arguments list is invalid, a
KeyError
exception will be raised.This class is the Python counterpart of the C++ class
pagmo::island
.- Keyword Arguments
udi – a user-defined island, either Python or C++
algo – a user-defined algorithm (either Python or C++), or an instance of
algorithm
pop (
population
) – a populationprob – a user-defined problem (either Python or C++), or an instance of
problem
b – a user-defined batch fitness evaluator (either Python or C++), or an instance of
bfe
size (
int
) – the number of individualsr_pol – a user-defined replacement policy (either Python or C++), or an instance of
r_policy
s_pol – a user-defined selection policy (either Python or C++), or an instance of
s_policy
seed (
int
) – the random seed (if not specified, it will be randomly-generated)
- Raises
KeyError – if the set of keyword arguments is invalid
unspecified – any exception thrown by the invoked C++ constructors, the deep copy of the UDI, the constructors of
algorithm
andpopulation
, failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- evolve(n=1)#
Launch evolution.
This method will evolve the island’s
population
using the island’salgorithm
. The evolution happens asynchronously: a call toevolve()
will create an evolution task that will be pushed to a queue, and then return immediately. The tasks in the queue are consumed by a separate thread of execution managed by theisland
object. Each task will invoke therun_evolve()
method of the UDI n times consecutively to perform the actual evolution. The island’s algorithm and population will be updated at the end of eachrun_evolve()
invocation. Exceptions raised inside the tasks are stored within the island object, and can be re-raised by callingwait_check()
.If the island is part of an
archipelago
, then migration of individuals to/from other islands might occur. The migration algorithm consists of the following steps:before invoking
run_evolve()
on the UDI, the island will ask the archipelago if there are candidate incoming individuals from other islands If so, the replacement policy is invoked and the current population of the island is updated with the migrants;run_evolve()
is then invoked and the current population is evolved;after
run_evolve()
has concluded, individuals are selected in the evolved population and copied into the migration database of the archipelago for future migrations.
It is possible to call this method multiple times to enqueue multiple evolution tasks, which will be consumed in a FIFO (first-in first-out) fashion. The user may call
wait()
orwait_check()
to block until all tasks have been completed, and to fetch exceptions raised during the execution of the tasks. Thestatus
attribute can be used to query the status of the asynchronous operations in the island.- Parameters
n (
int
) – the number of times therun_evolve()
method of the UDI will be called within the evolution task (this corresponds also to the number of times migration can happen, if the island belongs to an archipelago)- Raises
IndexError – if the island is part of an archipelago and during migration an invalid island index is used (this can happen if the archipelago’s topology is malformed)
OverflowError – if n is negative or larger than an implementation-defined value
unspecified – any exception thrown by the public interface of
archipelago
, the public interface of the replacement/selection policies, the underlying C++ method, or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- extract(t)#
Extract the user-defined island.
This method allows to extract a reference to the user-defined island (UDI) stored within this
island
instance. The behaviour of this function depends on the value of t (which must be atype
) and on the type of the internal UDI:if the type of the UDI is t, then a reference to the UDI will be returned (this mirrors the behaviour of the corresponding C++ method
pagmo::island::extract()
),if t is
object
and the UDI is a Python object (as opposed to an exposed C++ island), then a reference to the UDI will be returned (this allows to extract a Python UDI without knowing its type),otherwise,
None
will be returned.
- Parameters
t (
type
) – the type of the user-defined island to extract- Returns
a reference to the internal user-defined island, or
None
if the extraction fails- Raises
Examples
>>> import pygmo as pg >>> i1 = pg.island(algo=pg.de(), prob=pg.rosenbrock(), size=20, udi=pg.thread_island()) >>> i1.extract(pg.thread_island) <pygmo.core.thread_island at 0x7f8e478e1210> >>> i1.extract(pg.mp_island) is None True >>> class isl: ... def run_evolve(self, algo, pop): ... return algo, pop >>> i2 = pg.island(algo=pg.de(), prob=pg.rosenbrock(), size=20, udi=isl()) >>> i2.extract(object) <__main__.isl at 0x7f8e478261d0> >>> i2.extract(isl) <__main__.isl at 0x7f8e478261d0> >>> i2.extract(pg.thread_island) is None True
- get_algorithm()#
Get the algorithm.
It is safe to call this method while the island is evolving.
- Returns
a copy of the island’s algorithm
- Return type
- Raises
unspecified – any exception thrown by the underlying C++ method
- get_extra_info()#
Island’s extra info.
If the UDI provides a
get_extra_info()
method, then this method will return the output of itsget_extra_info()
method. Otherwise, an empty string will be returned.It is safe to call this method while the island is evolving.
- Returns
extra info about the UDI
- Return type
- Raises
unspecified – any exception thrown by the
get_extra_info()
method of the UDI
- get_name()#
Island’s name.
If the UDI provides a
get_name()
method, then this method will return the output of itsget_name()
method. Otherwise, an implementation-defined name based on the type of the UDI will be returned.It is safe to call this method while the island is evolving.
- Returns
the name of the UDI
- Return type
- Raises
unspecified – any exception thrown by the
get_name()
method of the UDI
- get_population()#
Get the population.
It is safe to call this method while the island is evolving.
- Returns
a copy of the island’s population
- Return type
- Raises
unspecified – any exception thrown by the underlying C++ method
- get_r_policy()#
Get the replacement policy.
- Returns
a copy of the current replacement policy
- Return type
- get_s_policy()#
Get the selection policy.
- Returns
a copy of the current selection policy
- Return type
- is_(t)#
Check the type of the user-defined island.
This method returns
False
ifextract(t)
returnsNone
, andTrue
otherwise.
- set_algorithm(algo)#
Set the algorithm.
It is safe to call this method while the island is evolving.
- Parameters
algo (
algorithm
) – the algorithm that will be copied into the island- Raises
unspecified – any exception thrown by the underlying C++ method
- set_population(pop)#
Set the population.
It is safe to call this method while the island is evolving.
- Parameters
pop (
population
) – the population that will be copied into the island- Raises
unspecified – any exception thrown by the underlying C++ method
- property status#
Status of the island.
This read-only property will return an
evolve_status
flag indicating the current status of asynchronous operations in the island. The flag will be:idle
if the island is currently not evolving and no exceptions were thrown by evolution tasks since the last call towait_check()
;busy
if the island is evolving and no exceptions have (yet) been thrown by evolution tasks since the last call towait_check()
;idle_error
if the island is currently not evolving and at least one evolution task threw an exception since the last call towait_check()
;busy_error
if the island is currently evolving and at least one evolution task has already thrown an exception since the last call towait_check()
.
Note that after a call to
wait_check()
,status
will always returnidle
, and after a call towait()
,status
will always return eitheridle
oridle_error
.- Returns
a flag indicating the current status of asynchronous operations in the island
- Return type
- wait()#
This method will block until all the evolution tasks enqueued via
evolve()
have been completed. Exceptions thrown by the enqueued tasks can be re-raised viawait_check()
: they will not be re-thrown by this method. Also, contrary towait_check()
, this method will not reset the status of the island: after a call towait()
,status
will always return either theidle
oridle_error
evolve_status
.
- wait_check()#
Block until evolution ends and re-raise the first stored exception.
If one task enqueued after the last call to
wait_check()
threw an exception, the exception will be re-thrown by this method. If more than one task enqueued after the last call towait_check()
threw an exception, this method will re-throw the exception raised by the first enqueued task that threw, and the exceptions from all the other tasks that threw will be ignored.Note that
wait_check()
resets the status of the island: after a call towait_check()
,status
will always return theidle
evolve_status
.- Raises
unspecified – any exception thrown by evolution tasks or by the underlying C++ method