Archipelago class#
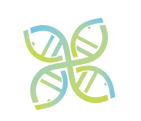
- class pygmo.archipelago(self, n=0, t=topology(), **kwargs)#
Archipelago.
An archipelago is a collection of
island
objects connected by atopology
. The islands in the archipelago can exchange individuals (i.e., candidate solutions) via a process called migration. The individuals migrate across the routes described by the topology, and the islands’ replacement and selection policies (seer_policy
ands_policy
) establish how individuals are replaced in and selected from the islands’ populations.The interface of
archipelago
mirrors partially the interface ofisland
: the evolution is initiated by a call toevolve()
, and at any time the user can query the state of the archipelago and access its island members. The user can explicitly wait for pending evolutions to conclude by calling thewait()
andwait_check()
methods. The status of ongoing evolutions in the archipelago can be queried viastatus()
.The constructor will initialise an archipelago with a topology t and n islands built from kwargs. The keyword arguments accept the same format as explained in the constructor of
island
, with the following differences:size is replaced by pop_size, for clarity,
the seed argument, if present, is used to initialise a random number generator that, in turn, is used to generate random seeds for each island population. In other words, the seed argument allows to generate randomly (but deterministically) the seeds of the populations in the archipelago. If seed is not provided, the seeds of the populations will be random and non-deterministic.
This class is the Python counterpart of the C++ class
pagmo::archipelago
.- Parameters
- Keyword Arguments
udi – a user-defined island, either Python or C++
algo – a user-defined algorithm (either Python or C++), or an instance of
algorithm
pop (
population
) – a populationprob – a user-defined problem (either Python or C++), or an instance of
problem
b – a user-defined batch fitness evaluator (either Python or C++), or an instance of
bfe
pop_size (
int
) – the number of individuals for each islandr_pol – a user-defined replacement policy (either Python or C++), or an instance of
r_policy
s_pol – a user-defined selection policy (either Python or C++), or an instance of
s_policy
seed (
int
) – the random seed
- Raises
TypeError – if n is not an integral type
ValueError – if n is negative
unspecified – any exception thrown by the constructor of
island
, by the underlying C++ constructor,push_back()
or by the public interface oftopology
Examples
>>> from pygmo import * >>> archi = archipelago(n = 16, algo = de(), prob = rosenbrock(10), pop_size = 20, seed = 32) >>> archi.evolve() >>> archi Number of islands: 16 Status: busy Islands summaries: # Type Algo Prob Size Status ----------------------------------------------------------------------------------------------- 0 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 1 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 2 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 3 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 4 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 busy 5 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 6 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 busy 7 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 busy 8 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 9 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 10 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 11 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 12 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle 13 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 busy 14 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 busy 15 Thread island Differential Evolution Multidimensional Rosenbrock Function 20 idle >>> archi.wait() >>> res = archi.get_champions_f() >>> res [array([ 125441.77328885]), array([ 144025.63904164]), array([ 25387.38989711]), array([ 56029.44160232]), array([ 47760.99082202]), array([ 118552.94891993]), array([ 118405.29575447]), array([ 101866.81846325]), array([ 166106.12039851]), array([ 167408.00058506]), array([ 148815.47953885]), array([ 165186.74476375]), array([ 326615.28881936]), array([ 167301.16445135]), array([ 166871.42760503]), array([ 75133.38815736])]
- evolve(n=1)#
Evolve archipelago.
This method will call
pygmo.island.evolve()
on all the islands of the archipelago. The input parameter n will be passed to the invocations ofpygmo.island.evolve()
for each island. Thestatus
attribute can be used to query the status of the asynchronous operations in the archipelago.- Parameters
n (
int
) – the parameter that will be passed topygmo.island.evolve()
- Raises
unspecified – any exception thrown by
pygmo.island.evolve()
- get_champions_f()#
Get the fitness vectors of the islands’ champions.
- Returns
the fitness vectors of the islands’ champions
- Return type
list
of 1D NumPy float arrays- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_champions_x()#
Get the decision vectors of the islands’ champions.
- Returns
the decision vectors of the islands’ champions
- Return type
list
of 1D NumPy float arrays- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_migrant_handling()#
- Returns
the current migrant handling policy for this archipelago
- Return type
- get_migrants_db()#
During the evolution of an archipelago, islands will periodically store the individuals selected for migration in a migrant database. This is a
list
oftuple
objects whose size is equal to the number of islands in the archipelago, and which contains the current candidate outgoing migrants for each island.The migrants tuples consist of 3 values each:
a 1D NumPy array of individual IDs (represented as 64-bit unsigned integrals),
a 2D NumPy array of decision vectors (i.e., the decision vectors of each individual, stored in row-major order),
a 2D NumPy array of fitness vectors (i.e., the fitness vectors of each individual, stored in row-major order).
- Returns
a copy of the database of migrants
- Return type
- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_migration_log()#
Each time an individual migrates from an island (the source) to another (the destination), an entry will be added to the migration log. The entry is a
tuple
of 6 elements containing:a timestamp of the migration,
the ID of the individual that migrated,
the decision and fitness vectors of the individual that migrated,
the indices of the source and destination islands.
The migration log is a
list
of migration entries.- Returns
a copy of the migration log
- Return type
- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_migration_type()#
- Returns
the current migration type for this archipelago
- Return type
- get_topology()#
- Returns
a copy of the current topology
- Return type
tyopology
- push_back(*args, **kwargs)#
Add an island.
This method will construct an island from the supplied arguments and add it to the archipelago. Islands are added at the end of the archipelago (that is, the new island will have an index equal to the size of the archipelago before the call to this method).
pygmo.topology.push_back()
will also be called on thetopology
associated to this archipelago, so that the addition of a new island to the archipelago is mirrored by the addition of a new vertex to the topology.This method accepts either a single positional argument, or a set of keyword arguments:
if no positional arguments are provided, then the keyword arguments will be used to construct a
island
which will then be added to the archipelago; otherwise,if a single positional argument is provided and no keyword arguments are provided, then the positional argument is interpreted as an
island
object to be added to the archipelago.
Any other combination of positional/keyword arguments will result in an error.
- Raises
ValueError – if, when using positional arguments, there are more than 1 positional arguments, or if keyword arguments are also used at the same time
TypeError – if, when using a single positional argument, the type of that argument is not
island
unspecified – any exception thrown by the constructor of
island
,pygmo.topology.push_back()
or by the underlying C++ method
- set_migrant_handling(mh)#
Set a new migrant handling policy for this archipelago.
- Parameters
mh (
migrant_handling
) – the desired migrant handling policy for this archipelago
- set_migrants_db(mig)#
During the evolution of an archipelago, islands will periodically store the individuals selected for migration in a migrant database. This is a
list
oftuple
objects whose size is equal to the number of islands in the archipelago, and which contains the current candidate outgoing migrants for each island.The migrants tuples consist of 3 values each:
a 1D NumPy array of individual IDs (represented as 64-bit unsigned integrals),
a 2D NumPy array of decision vectors (i.e., the decision vectors of each individual, stored in row-major order),
a 2D NumPy array of fitness vectors (i.e., the fitness vectors of each individual, stored in row-major order).
This setter allows to replace the current database of migrants with a new one.
Note that this setter will accept in input a malformed database of migrants without complaining. An invalid database of migrants will however result in exceptions being raised when migration occurs.
- Parameters
mig (
list
) – the new database of migrants- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- set_migration_type(mt)#
Set a new migration type for this archipelago.
- Parameters
mt (
migration_type
) – the desired migration type for this archipelago
- set_topology(t)#
This method will wait for any ongoing evolution in the archipelago to finish, and it will then set the topology of the archipelago to t.
Note that it is the user’s responsibility to ensure that the new topology is consistent with the archipelago’s properties.
- Parameters
t – a user-defined topology (either Python or C++), or an instance of
topology
- Raises
unspecified – any exception thrown by copying the topology
- property status#
Status of the archipelago.
This read-only property will return an
evolve_status
flag indicating the current status of asynchronous operations in the archipelago. The flag will be:idle
if, for all the islands in the archipelago,pygmo.island.status
returnsidle
;busy
if, for at least one island in the archipelago,pygmo.island.status
returnsbusy
, and for no islandpygmo.island.status
returns an error status;idle_error
if no island in the archipelago is busy and for at least one islandpygmo.island.status
returnsidle_error
;busy_error
if, for at least one island in the archipelago,pygmo.island.status
returns an error status and at least one island is busy.
Note that after a call to
wait_check()
,pygmo.archipelago.status
will always returnidle
, and after a call towait()
,pygmo.archipelago.status
will always return eitheridle
oridle_error
.- Returns
a flag indicating the current status of asynchronous operations in the archipelago
- Return type
- wait()#
Block until all evolutions have finished.
This method will call
pygmo.island.wait()
on all the islands of the archipelago. Exceptions thrown by island evolutions can be re-raised viawait_check()
: they will not be re-thrown by this method. Also, contrary towait_check()
, this method will not reset the status of the archipelago: after a call towait()
, thestatus
attribute will always return eitheridle
oridle_error
.
- wait_check()#
Block until all evolutions have finished and raise the first exception that was encountered.
This method will call
pygmo.island.wait_check()
on all the islands of the archipelago (following the order in which the islands were inserted into the archipelago). The first exception raised bypygmo.island.wait_check()
will be re-raised by this method, and all the exceptions thrown by the other calls topygmo.island.wait_check()
will be ignored.Note that
wait_check()
resets the status of the archipelago: after a call towait_check()
, thestatus
attribute will always returnidle
.- Raises
unspecified – any exception thrown by any evolution task queued in the archipelago’s islands