Problem class#
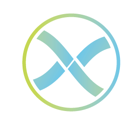
- class pygmo.problem(udp=null_problem())#
Problem class.
This class represents a generic mathematical programming or evolutionary optimization problem in the form:
\[\begin{split}\begin{array}{rl} \mbox{find:} & \mathbf {lb} \le \mathbf x \le \mathbf{ub}\\ \mbox{to minimize: } & \mathbf f(\mathbf x, s) \in \mathbb R^{n_{obj}}\\ \mbox{subject to:} & \mathbf {c}_e(\mathbf x, s) = 0 \\ & \mathbf {c}_i(\mathbf x, s) \le 0 \end{array}\end{split}\]where \(\mathbf x \in \mathbb R^{n_{cx}} \times \mathbb Z^{n_{ix}}\) is called decision vector or chromosome, and is made of \(n_{cx}\) real numbers and \(n_{ix}\) integers (all represented as doubles). The total problem dimension is then indicated with \(n_x = n_{cx} + n_{ix}\). \(\mathbf{lb}, \mathbf{ub} \in \mathbb R^{n_{cx}} \times \mathbb Z^{n_{ix}}\) are the box-bounds, \(\mathbf f: \mathbb R^{n_{cx}} \times \mathbb Z^{n_{ix}} \rightarrow \mathbb R^{n_{obj}}\) define the objectives, \(\mathbf c_e: \mathbb R^{n_{cx}} \times \mathbb Z^{n_{ix}} \rightarrow \mathbb R^{n_{ec}}\) are non linear equality constraints, and \(\mathbf c_i: \mathbb R^{n_{cx}} \times \mathbb Z^{n_{ix}} \rightarrow \mathbb R^{n_{ic}}\) are non linear inequality constraints. Note that the objectives and constraints may also depend from an added value \(s\) seeding the values of any number of stochastic variables. This allows also for stochastic programming tasks to be represented by this class. A tolerance is also considered for all constraints and set, by default, to zero. It can be modified via the
c_tol
attribute.In order to define an optimizaztion problem in pygmo, the user must first define a class whose methods describe the properties of the problem and allow to compute the objective function, the gradient, the constraints, etc. In pygmo, we refer to such a class as a user-defined problem, or UDP for short. Once defined and instantiated, a UDP can then be used to construct an instance of this class,
problem
, which provides a generic interface to optimization problems.Every UDP must implement at least the following two methods:
def fitness(self, dv): ... def get_bounds(self): ...
The
fitness()
method is expected to return the fitness of the input decision vector (concatenating the objectives, the equality and the inequality constraints), whileget_bounds()
is expected to return the box bounds of the problem, \((\mathbf{lb}, \mathbf{ub})\), which also implicitly define the dimension of the problem. Thefitness()
andget_bounds()
methods of the UDP are accessible from the correspondingpygmo.problem.fitness()
andpygmo.problem.get_bounds()
methods (see their documentation for information on how the two methods should be implemented in the UDP and other details).The two mandatory methods above allow to define a single objective, deterministic, derivative-free, unconstrained optimization problem. In order to consider more complex cases, the UDP may implement one or more of the following methods:
def get_nobj(self): ... def get_nec(self): ... def get_nic(self): ... def get_nix(self): ... def batch_fitness(self, dvs): ... def has_batch_fitness(self): ... def has_gradient(self): ... def gradient(self, dv): ... def has_gradient_sparsity(self): ... def gradient_sparsity(self): ... def has_hessians(self): ... def hessians(self, dv): ... def has_hessians_sparsity(self): ... def hessians_sparsity(self): ... def has_set_seed(self): ... def set_seed(self, s): ... def get_name(self): ... def get_extra_info(self): ...
See the documentation of the corresponding methods in this class for details on how the optional methods in the UDP should be implemented and on how they are used by
problem
. Note that the exposed C++ problems can also be used as UDPs, even if they do not expose any of the mandatory or optional methods listed above (see here for the full list of UDPs already coded in pygmo).This class is the Python counterpart of the C++ class
pagmo::problem
.- Parameters
udp – a user-defined problem, either C++ or Python
- Raises
NotImplementedError – if udp does not implement the mandatory methods detailed above
ValueError – if the number of objectives of the UDP is zero, the number of objectives, equality or inequality constraints is larger than an implementation-defined value, the problem bounds are invalid (e.g., they contain NaNs, the dimensionality of the lower bounds is different from the dimensionality of the upper bounds, etc. - note that infinite bounds are allowed), or if the
gradient_sparsity()
andhessians_sparsity()
methods of the UDP fail basic sanity checks (e.g., they return vectors with repeated indices, they contain indices exceeding the problem’s dimensions, etc.)unspecified – any exception thrown by methods of the UDP invoked during construction, the deep copy of the UDP, the constructor of the underlying C++ class, failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- batch_fitness(dvs)#
This method implements the evaluation of multiple decision vectors in batch mode by invoking the
batch_fitness()
method of the UDP. Thebatch_fitness()
method of the UDP accepts in input a batch of decision vectors, dvs, stored contiguously: for a problem with dimension \(n\), the first decision vector in dvs occupies the index range \(\left[0, n\right)\), the second decision vector occupies the range \(\left[n, 2n\right)\), and so on. The return value is the batch of fitness vectors fvs resulting from computing the fitness of the input decision vectors. fvs is also stored contiguously: for a problem with fitness dimension \(f\), the first fitness vector will occupy the index range \(\left[0, f\right)\), the second fitness vector will occupy the range \(\left[f, 2f\right)\), and so on.If the UDP provides a
batch_fitness()
method, this method will forwarddvs
to thebatch_fitness()
method of the UDP after sanity checks. The output of thebatch_fitness()
method of the UDP will also be checked before being returned. If the UDP does not provide abatch_fitness()
method, an error will be raised.A successful call of this method will increase the internal fitness evaluation counter (see
get_fevals()
).The
batch_fitness()
method of the UDP must be able to take as input the decision vectors as a 1D NumPy array, and it must return the fitness vectors as an iterable Python object (e.g., 1D NumPy array, list, tuple, etc.).- Parameters
dvs (array-like object) – the decision vectors (chromosomes) to be evaluated in batch mode
- Returns
the fitness vectors of dvs
- Return type
1D NumPy float array
- Raises
ValueError – if dvs and/or the return value are not compatible with the problem’s properties
unspecified – any exception thrown by the
batch_fitness()
method of the UDP, or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- property c_tol#
Constraints tolerance.
This property contains an array of
float
that are used when checking for constraint feasibility. The dimension of the array is \(n_{ec} + n_{ic}\) (i.e., the total number of constraints), and the array is zero-filled on problem construction.This property can also be set via a scalar, instead of an array. In such case, all the tolerances will be set to the provided scalar value.
- Returns
the constraints’ tolerances
- Return type
1D NumPy float array
- Raises
ValueError – if, when setting this property, the size of the input array differs from the number of constraints of the problem or if any element of the array is negative or NaN
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
Examples
>>> from pygmo import problem, hock_schittkowski_71 as hs71 >>> prob = problem(hs71()) >>> prob.c_tol array([0., 0.]) >>> prob.c_tol = [1, 2] >>> prob.c_tol array([1., 2.]) >>> prob.c_tol = .5 >>> prob.c_tol array([0.5, 0.5])
- extract(t)#
Extract the user-defined problem.
This method allows to extract a reference to the user-defined problem (UDP) stored within this
problem
instance. The behaviour of this function depends on the value of t (which must be atype
) and on the type of the internal UDP:if the type of the UDP is t, then a reference to the UDP will be returned (this mirrors the behaviour of the corresponding C++ method
pagmo::problem::extract()
),if t is
object
and the UDP is a Python object (as opposed to an exposed C++ problem), then a reference to the UDP will be returned (this allows to extract a Python UDP without knowing its type),otherwise,
None
will be returned.
- Parameters
t (
type
) – the type of the user-defined problem to extract- Returns
a reference to the internal user-defined problem, or
None
if the extraction fails- Raises
Examples
>>> import pygmo as pg >>> p1 = pg.problem(pg.rosenbrock()) >>> p1.extract(pg.rosenbrock) <pygmo.core.rosenbrock at 0x7f56b870fd50> >>> p1.extract(pg.ackley) is None True >>> class prob: ... def fitness(self, x): ... return [x[0]] ... def get_bounds(self): ... return ([0],[1]) >>> p2 = pg.problem(prob()) >>> p2.extract(object) <__main__.prob at 0x7f56a66b6588> >>> p2.extract(prob) <__main__.prob at 0x7f56a66b6588> >>> p2.extract(pg.rosenbrock) is None True
- feasibility_f(f)#
This method will check the feasibility of a fitness vector f against the tolerances returned by
c_tol
.- Parameters
f (array-like object) – a fitness vector
- Returns
True
if the fitness vector is feasible,False
otherwise- Return type
bool
- Raises
ValueError – if the size of f is not the same as the output of
get_nf()
- feasibility_x(x)#
This method will check the feasibility of the fitness corresponding to a decision vector x against the tolerances returned by
c_tol
.This will cause one fitness evaluation.
- Parameters
dv (array-like object) – a decision vector
- Returns
True
if x results in a feasible fitness,False
otherwise- Return type
bool
- Raises
unspecified – any exception thrown by
feasibility_f()
orfitness()
- fitness(dv)#
Fitness.
This method will invoke the
fitness()
method of the UDP to compute the fitness of the input decision vector dv. The return value of thefitness()
method of the UDP is expected to have a dimension of \(n_{f} = n_{obj} + n_{ec} + n_{ic}\) and to contain the concatenated values of \(\mathbf f, \mathbf c_e\) and \(\mathbf c_i\) (in this order). Equality constraints are all assumed in the form \(c_{e_i}(\mathbf x) = 0\) while inequalities are assumed in the form \(c_{i_i}(\mathbf x) <= 0\) so that negative values are associated to satisfied inequalities.In addition to invoking the
fitness()
method of the UDP, this method will perform sanity checks on dv and on the returned fitness vector. A successful call of this method will increase the internal fitness evaluation counter (seeget_fevals()
).The
fitness()
method of the UDP must be able to take as input the decision vector as a 1D NumPy array, and it must return the fitness vector as an iterable Python object (e.g., 1D NumPy array, list, tuple, etc.).- Parameters
dv (array-like object) – the decision vector (chromosome) to be evaluated
- Returns
the fitness of dv
- Return type
1D NumPy float array
- Raises
ValueError – if either the length of dv differs from the value returned by
get_nx()
, or the length of the returned fitness vector differs from the value returned byget_nf()
unspecified – any exception thrown by the
fitness()
method of the UDP, or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_bounds()#
Box-bounds.
This method will return the box-bounds \((\mathbf{lb}, \mathbf{ub})\) of the problem, as returned by the
get_bounds()
method of the UDP. Infinities in the bounds are allowed.The
get_bounds()
method of the UDP must return the box-bounds as a tuple of 2 elements, the lower bounds vector and the upper bounds vector, which must be represented as iterable Python objects (e.g., 1D NumPy arrays, lists, tuples, etc.). The box-bounds returned by the UDP are checked upon the construction of aproblem
.- Returns
a tuple of two 1D NumPy float arrays representing the lower and upper box-bounds of the problem
- Return type
- Raises
unspecified – any exception thrown by the invoked method of the underlying C++ class, or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_extra_info()#
Problem’s extra info.
If the UDP provides a
get_extra_info()
method, then this method will return the output of itsget_extra_info()
method. Otherwise, an empty string will be returned.- Returns
extra info about the UDP
- Return type
- Raises
unspecified – any exception thrown by the
get_extra_info()
method of the UDP
- get_fevals()#
Number of fitness evaluations.
Each time a call to
fitness()
successfully completes, an internal counter is increased by one. The counter is initialised to zero upon problem construction and it is never reset. Copy operations copy the counter as well.
- get_gevals()#
Number of gradient evaluations.
Each time a call to
gradient()
successfully completes, an internal counter is increased by one. The counter is initialised to zero upon problem construction and it is never reset. Copy operations copy the counter as well.- Returns
the number of times
gradient()
was successfully called- Return type
- get_hevals()#
Number of hessians evaluations.
Each time a call to
hessians()
successfully completes, an internal counter is increased by one. The counter is initialised to zero upon problem construction and it is never reset. Copy operations copy the counter as well.- Returns
the number of times
hessians()
was successfully called- Return type
- get_lb()#
Lower box-bounds.
This method will return the lower box-bounds for this problem. See
get_bounds()
for a detailed explanation of how the bounds are determined.- Returns
an array representing the lower box-bounds of this problem
- Return type
1D NumPy float array
- Raises
unspecified – any exception thrown by the invoked method of the underlying C++ class, or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- get_name()#
Problem’s name.
If the UDP provides a
get_name()
method, then this method will return the output of itsget_name()
method. Otherwise, an implementation-defined name based on the type of the UDP will be returned.- Returns
the problem’s name
- Return type
- get_nc()#
Total number of constraints.
This method will return the sum of the output of
get_nic()
andget_nec()
(i.e., the total number of constraints).- Returns
the total number of constraints of the problem
- Return type
- get_ncx()#
Continuous dimension of the problem.
This method will return \(n_{cx}\), the continuous dimension of the problem.
- Returns
the continuous dimension of the problem
- Return type
- get_nec()#
Number of equality constraints.
This method will return \(n_{ec}\), the number of equality constraints of the problem.
The optional
get_nec()
method of the UDP must return the number of equality constraints as anint
. If the UDP does not implement theget_nec()
method, zero equality constraints will be assumed. The number of equality constraints returned by the UDP is checked upon the construction of aproblem
.- Returns
the number of equality constraints of the problem
- Return type
- get_nf()#
Dimension of the fitness.
This method will return \(n_{f}\), the dimension of the fitness, which is the sum of \(n_{obj}\), \(n_{ec}\) and \(n_{ic}\).
- Returns
the dimension of the fitness
- Return type
- get_nic()#
Number of inequality constraints.
This method will return \(n_{ic}\), the number of inequality constraints of the problem.
The optional
get_nic()
method of the UDP must return the number of inequality constraints as anint
. If the UDP does not implement theget_nic()
method, zero inequality constraints will be assumed. The number of inequality constraints returned by the UDP is checked upon the construction of aproblem
.- Returns
the number of inequality constraints of the problem
- Return type
- get_nix()#
Integer dimension of the problem.
This method will return \(n_{ix}\), the integer dimension of the problem.
The optional
get_nix()
method of the UDP must return the problem’s integer dimension as anint
. If the UDP does not implement theget_nix()
method, a zero integer dimension will be assumed. The integer dimension returned by the UDP is checked upon the construction of aproblem
.- Returns
the integer dimension of the problem
- Return type
- get_nobj()#
Number of objectives.
This method will return \(n_{obj}\), the number of objectives of the problem.
The optional
get_nobj()
method of the UDP must return the number of objectives as anint
. If the UDP does not implement theget_nobj()
method, a single-objective optimizaztion problem will be assumed. The number of objectives returned by the UDP is checked upon the construction of aproblem
.- Returns
the number of objectives of the problem
- Return type
- get_nx()#
Dimension of the problem.
This method will return \(n_{x}\), the dimension of the problem as established by the length of the bounds returned by
get_bounds()
.- Returns
the dimension of the problem
- Return type
- get_thread_safety()#
Problem’s thread safety level.
This method will return a value of the enum
pygmo.thread_safety
which indicates the thread safety level of the UDP. Unlike in C++, in Python it is not possible to re-implement this method in the UDP. That is, for C++ UDPs, the returned value will be the value returned by theget_thread_safety()
method of the UDP. For Python UDPs, the returned value will be unconditionallynone
.- Returns
the thread safety level of the UDP
- Return type
a value of
pygmo.thread_safety
- get_ub()#
Upper box-bounds.
This method will return the upper box-bounds for this problem. See
get_bounds()
for a detailed explanation of how the bounds are determined.- Returns
an array representing the upper box-bounds of this problem
- Return type
1D NumPy float array
- Raises
unspecified – any exception thrown by the invoked method of the underlying C++ class, or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- gradient(dv)#
Gradient.
This method will compute the gradient of the input decision vector dv by invoking the
gradient()
method of the UDP. Thegradient()
method of the UDP must return a sparse representation of the gradient: the \(k\)-th term of the gradient vector is expected to contain \(\frac{\partial f_i}{\partial x_j}\), where the pair \((i,j)\) is the \(k\)-th element of the sparsity pattern (collection of index pairs), as returned bygradient_sparsity()
.If the UDP provides a
gradient()
method, this method will forward dv to thegradient()
method of the UDP after sanity checks. The output of thegradient()
method of the UDP will also be checked before being returned. If the UDP does not provide agradient()
method, an error will be raised. A successful call of this method will increase the internal gradient evaluation counter (seeget_gevals()
).The
gradient()
method of the UDP must be able to take as input the decision vector as a 1D NumPy array, and it must return the gradient vector as an iterable Python object (e.g., 1D NumPy array, list, tuple, etc.).- Parameters
dv (array-like object) – the decision vector whose gradient will be computed
- Returns
the gradient of dv
- Return type
1D NumPy float array
- Raises
ValueError – if either the length of dv differs from the value returned by
get_nx()
, or the returned gradient vector does not have the same size as the vector returned bygradient_sparsity()
NotImplementedError – if the UDP does not provide a
gradient()
methodunspecified – any exception thrown by the
gradient()
method of the UDP, or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- gradient_sparsity()#
Gradient sparsity pattern.
This method will return the gradient sparsity pattern of the problem. The gradient sparsity pattern is a lexicographically sorted collection of the indices \((i,j)\) of the non-zero elements of \(g_{ij} = \frac{\partial f_i}{\partial x_j}\).
If
has_gradient_sparsity()
returnsTrue
, then thegradient_sparsity()
method of the UDP will be invoked, and its result returned (after sanity checks). Otherwise, a a dense pattern is assumed and the returned vector will be \(((0,0),(0,1), ... (0,n_x-1), ...(n_f-1,n_x-1))\).The
gradient_sparsity()
method of the UDP must return either a 2D NumPy array of integers, or an iterable Python object of any kind. Specifically:if the returned value is a NumPy array, its shape must be \((n,2)\) (with \(n \geq 0\)),
if the returned value is an iterable Python object, then its elements must in turn be iterable Python objects containing each exactly 2 elements representing the indices \((i,j)\).
- Returns
the gradient sparsity pattern
- Return type
2D Numpy int array
- Raises
ValueError – if the NumPy array returned by the UDP does not satisfy the requirements described above (e.g., invalid shape, dimensions, etc.), at least one element of the returned iterable Python object does not consist of a collection of exactly 2 elements, or the sparsity pattern returned by the UDP is invalid (specifically, if it is not strictly sorted lexicographically, or if the indices in the pattern are incompatible with the properties of the problem, or if the size of the returned pattern is different from the size recorded upon construction)
OverflowError – if the NumPy array returned by the UDP contains integer values which are negative or outside an implementation-defined range
unspecified – any exception thrown by the underlying C++ function, the
PyArray_FROM_OTF()
function from the NumPy C API, or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- has_batch_fitness()#
Check if the
batch_fitness()
method is available in the UDP.This method will return
True
if thebatch_fitness()
method is available in the UDP,False
otherwise.The availability of the
batch_fitness()
method is determined as follows:if the UDP does not provide a
batch_fitness()
method, then this method will always returnFalse
;if the UDP provides a
batch_fitness()
method but it does not provide ahas_batch_fitness()
method, then this method will always returnTrue
;if the UDP provides both a
batch_fitness()
and ahas_batch_fitness()
method, then this method will return the output of thehas_batch_fitness()
method of the UDP.
The optional
has_batch_fitness()
method of the UDP must return abool
. For information on how to implement thebatch_fitness()
method of the UDP, seebatch_fitness()
.- Returns
a flag signalling the availability of the
batch_fitness()
method in the UDP- Return type
bool
- has_gradient()#
Check if the gradient is available in the UDP.
This method will return
True
if the gradient is available in the UDP,False
otherwise.The availability of the gradient is determined as follows:
if the UDP does not provide a
gradient()
method, then this method will always returnFalse
;if the UDP provides a
gradient()
method but it does not provide ahas_gradient()
method, then this method will always returnTrue
;if the UDP provides both a
gradient()
and ahas_gradient()
method, then this method will return the output of thehas_gradient()
method of the UDP.
The optional
has_gradient()
method of the UDP must return abool
. For information on how to implement thegradient()
method of the UDP, seegradient()
.- Returns
a flag signalling the availability of the gradient in the UDP
- Return type
bool
- has_gradient_sparsity()#
Check if the gradient sparsity is available in the UDP.
This method will return
True
if the gradient sparsity is available in the UDP,False
otherwise.The availability of the gradient sparsity is determined as follows:
if the UDP does not provide a
gradient_sparsity()
method, then this method will always returnFalse
;if the UDP provides a
gradient_sparsity()
method but it does not provide ahas_gradient_sparsity()
method, then this method will always returnTrue
;if the UDP provides both a
gradient_sparsity()
method and ahas_gradient_sparsity()
method, then this method will return the output of thehas_gradient_sparsity()
method of the UDP.
The optional
has_gradient_sparsity()
method of the UDP must return abool
. For information on how to implement thegradient_sparsity()
method of the UDP, seegradient_sparsity()
.Note
Regardless of what this method returns, the
gradient_sparsity()
method will always return a sparsity pattern: if the UDP does not provide the gradient sparsity, pygmo will assume that the sparsity pattern of the gradient is dense. Seegradient_sparsity()
for more details.- Returns
a flag signalling the availability of the gradient sparsity in the UDP
- Return type
bool
- has_hessians()#
Check if the hessians are available in the UDP.
This method will return
True
if the hessians are available in the UDP,False
otherwise.The availability of the hessians is determined as follows:
if the UDP does not provide a
hessians()
method, then this method will always returnFalse
;if the UDP provides a
hessians()
method but it does not provide ahas_hessians()
method, then this method will always returnTrue
;if the UDP provides both a
hessians()
and ahas_hessians()
method, then this method will return the output of thehas_hessians()
method of the UDP.
The optional
has_hessians()
method of the UDP must return abool
. For information on how to implement thehessians()
method of the UDP, seehessians()
.- Returns
a flag signalling the availability of the hessians in the UDP
- Return type
bool
- has_hessians_sparsity()#
Check if the hessians sparsity is available in the UDP.
This method will return
True
if the hessians sparsity is available in the UDP,False
otherwise.The availability of the hessians sparsity is determined as follows:
if the UDP does not provide a
hessians_sparsity()
method, then this method will always returnFalse
;if the UDP provides a
hessians_sparsity()
method but it does not provide ahas_hessians_sparsity()
method, then this method will always returnTrue
;if the UDP provides both a
hessians_sparsity()
method and ahas_hessians_sparsity()
method, then this method will return the output of thehas_hessians_sparsity()
method of the UDP.
The optional
has_hessians_sparsity()
method of the UDP must return abool
. For information on how to implement thehessians_sparsity()
method of the UDP, seehessians_sparsity()
.Note
Regardless of what this method returns, the
hessians_sparsity()
method will always return a sparsity pattern: if the UDP does not provide the hessians sparsity, pygmo will assume that the sparsity pattern of the hessians is dense. Seehessians_sparsity()
for more details.- Returns
a flag signalling the availability of the hessians sparsity in the UDP
- Return type
bool
- has_set_seed()#
Check if the
set_seed()
method is available in the UDP.This method will return
True
if theset_seed()
method is available in the UDP,False
otherwise.The availability of the
set_seed()
method is determined as follows:if the UDP does not provide a
set_seed()
method, then this method will always returnFalse
;if the UDP provides a
set_seed()
method but it does not provide ahas_set_seed()
method, then this method will always returnTrue
;if the UDP provides both a
set_seed()
and ahas_set_seed()
method, then this method will return the output of thehas_set_seed()
method of the UDP.
The optional
has_set_seed()
method of the UDP must return abool
. For information on how to implement theset_seed()
method of the UDP, seeset_seed()
.- Returns
a flag signalling the availability of the
set_seed()
method in the UDP- Return type
bool
- hessians(dv)#
Hessians.
This method will compute the hessians of the input decision vector dv by invoking the
hessians()
method of the UDP. Thehessians()
method of the UDP must return a sparse representation of the hessians: the element \(l\) of the returned vector contains \(h^l_{ij} = \frac{\partial f^2_l}{\partial x_i\partial x_j}\) in the order specified by the \(l\)-th element of the hessians sparsity pattern (a vector of index pairs \((i,j)\)) as returned byhessians_sparsity()
. Since the hessians are symmetric, their sparse representation contains only lower triangular elements.If the UDP provides a
hessians()
method, this method will forward dv to thehessians()
method of the UDP after sanity checks. The output of thehessians()
method of the UDP will also be checked before being returned. If the UDP does not provide ahessians()
method, an error will be raised. A successful call of this method will increase the internal hessians evaluation counter (seeget_hevals()
).The
hessians()
method of the UDP must be able to take as input the decision vector as a 1D NumPy array, and it must return the hessians vector as an iterable Python object (e.g., list, tuple, etc.).- Parameters
dv (array-like object) – the decision vector whose hessians will be computed
- Returns
the hessians of dv
- Return type
list
of 1D NumPy float array- Raises
ValueError – if the length of dv differs from the value returned by
get_nx()
, or the length of returned hessians does not match the corresponding hessians sparsity pattern dimensions, or the size of the return value is not equal to the fitness dimensionNotImplementedError – if the UDP does not provide a
hessians()
methodunspecified – any exception thrown by the
hessians()
method of the UDP, or by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- hessians_sparsity()#
Hessians sparsity pattern.
This method will return the hessians sparsity pattern of the problem. Each component \(l\) of the hessians sparsity pattern is a lexicographically sorted collection of the indices \((i,j)\) of the non-zero elements of \(h^l_{ij} = \frac{\partial f^l}{\partial x_i\partial x_j}\). Since the Hessian matrix is symmetric, only lower triangular elements are allowed.
If
has_hessians_sparsity()
returnsTrue
, then thehessians_sparsity()
method of the UDP will be invoked, and its result returned (after sanity checks). Otherwise, a dense pattern is assumed and \(n_f\) sparsity patterns containing \(((0,0),(1,0), (1,1), (2,0) ... (n_x-1,n_x-1))\) will be returned.The
hessians_sparsity()
method of the UDP must return an iterable Python object of any kind. Each element of the returned object will then be interpreted as a sparsity pattern in the same way as described ingradient_sparsity()
. Specifically:if the element is a NumPy array, its shape must be \((n,2)\) (with \(n \geq 0\)),
if the element is itself an iterable Python object, then its elements must in turn be iterable Python objects containing each exactly 2 elements representing the indices \((i,j)\).
- Returns
the hessians sparsity patterns
- Return type
list
of 2D Numpy int array- Raises
ValueError – if the NumPy arrays returned by the UDP do not satisfy the requirements described above (e.g., invalid shape, dimensions, etc.), at least one element of a returned iterable Python object does not consist of a collection of exactly 2 elements, or if a sparsity pattern returned by the UDP is invalid (specifically, if it is not strictly sorted lexicographically, if the indices in the pattern are incompatible with the properties of the problem or if the size of the pattern differs from the size recorded upon construction)
OverflowError – if the NumPy arrays returned by the UDP contain integer values which are negative or outside an implementation-defined range
unspecified – any exception thrown by the underlying C++ function, the
PyArray_FROM_OTF()
function from the NumPy C API, or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- increment_fevals(n)#
Increment the number of fitness evaluations.
New in version 2.13.
This method will increase the internal counter of fitness evaluations by n.
- Parameters
n (
int
) – the amount by which the internal counter of fitness evaluations will be increased- Raises
unspecified – any exception thrown by failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)
- is_(t)#
Check the type of the user-defined problem.
This method returns
False
ifextract(t)
returnsNone
, andTrue
otherwise.
- is_stochastic()#
Alias for
has_set_seed()
.
- set_seed(seed)#
Set the seed for the stochastic variables.
This method will set the seed to be used in the fitness function to instantiate all stochastic variables. If the UDP provides a
set_seed()
method, then itsset_seed()
method will be invoked. Otherwise, an error will be raised. The seed parameter must be non-negative.The
set_seed()
method of the UDP must be able to take anint
as input parameter.- Parameters
seed (
int
) – the desired seed value- Raises
NotImplementedError – if the UDP does not provide a
set_seed()
methodOverflowError – if seed is negative
unspecified – any exception raised by the
set_seed()
method of the UDP or failures at the intersection between C++ and Python (e.g., type conversion errors, mismatched function signatures, etc.)